C#7 and tuples
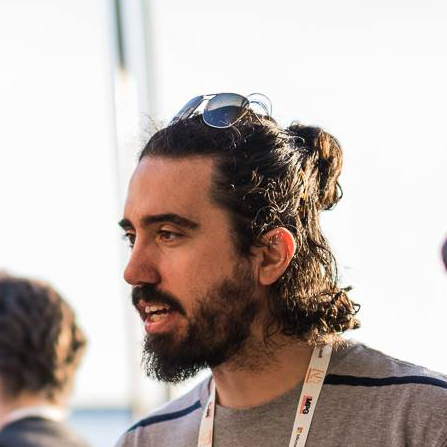
C# 7 is fun! Tuples are awesome and allow one to quickly swap variables:
(x, y) = (y, x);
Not only that, let's say you have the following class:
public class Vector2
{
public int X { get; }
public int Y { get; }
public Vector2(int x, int y)
{
X = x;
Y = y;
}
}
The constructor can be simplified to the following:
public Vector2(int x, int y) => (X, Y) = (x, y);
One can even play with fluent validation:
public struct Message
{
public string Text { get; }
public string Sender { get; }
public Message(string text, string sender)
=> (Text, Sender) = (text.EnsureNotNull(), sender ?? string.Empty);
}
public static class Extensions
{
public static T EnsureNotNull<T>(this T o) where T : class
=> o is null
? throw new ArgumentNullException()
: o;
}
Notice the use of new throw expressions feature.